When it comes to WordPress, with currently 48,413 plugins out there, there is a good chance you will find a good fit for your needs in the official plugins directory ( https://wordpress.org/plugins/ ). There are times, however, when you’ll need a completely bespoke solution to add functionality to your site.
In this article, I’ll go through the basics of how to create a clean, simple and object oriented architecture for any kind of WordPress plugin.
Main file
To create a WordPress plugin, you only need one PHP file with a specific header comment:
<?php /** * Plugin Name: Awesome Plugin */
This file should be placed in the wp-content/plugins folder uniquely named as the plugin itself, in our case, it will be awesome-plugin.php.
It is recommended to also place this file within another folder named as well after your plugin, this will help organize future files such as assets, JavaScript or CSS.
Once this file is created you should be able to locate your new plugin in your WordPress admin and enable it. Of course, it won’t do anything, just yet!
You can potentially put all your code in this file, however, that will make your life (or the next developer’s) harder, especially when you start having thousands of lines of unorganized code.
A better way is to have a small class, with the only purpose to define the plugin itself and its global settings. A good use would be declaring the plugin main hooks in there, like the activation, deactivation and uninstall ones.
<?php //Define Dirpath for hooks define( 'DIR_PATH', plugin_dir_path( __FILE__ ) ); /** * Plugin Name: Awesome Plugin * Version: 1.0 * Description: This is the best plugin! * Author: Andrea Fuggetta <contact@ndevr.io> * Author URI: https://www.ndevr.io * Plugin URI: https://www.ndevr.io */ if ( ! class_exists( 'AwesomePlugin' ) ) { class AwesomePlugin { /** * Constructor */ public function __construct() { $this->setup_actions(); } /** * Setting up Hooks */ public function setup_actions() { //Main plugin hooks register_activation_hook( DIR_PATH, array( 'AwesomePlugin', 'activate' ) ); register_deactivation_hook( DIR_PATH, array( 'AwesomePlugin', 'deactivate' ) ); } /** * Activate callback */ public static function activate() { //Activation code in here } /** * Deactivate callback */ public static function deactivate() { //Deactivation code in here } } // instantiate the plugin class $wp_plugin_template = new AwesomePlugin(); }
File structure
Chances are your plugin will have front facing widgets or pages that will need to be styled, or some additional JavaScript functionality to be added. Where would you put these?
A fitting place would be in an asset directory including js and CSS folders which will contain relevant files. You can also go a step further and add src and build sub-folders containing respectively non-minified/non-compressed files and production ready files.
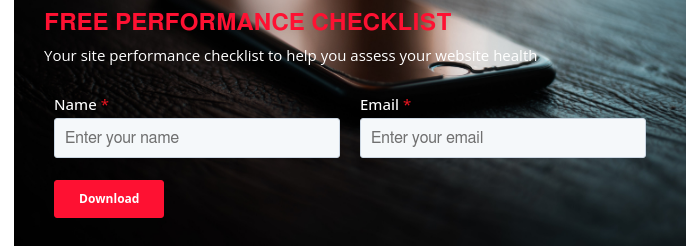
Depending on the magnitude of your plugin you may need to use more files to enhance functionality or simply sub-divide pre-existing one. A good way of doing this is to create another folder called inc ( or lib ). Each file contained in this folder should be in its relevant namespace ( if you are not familiar with namespaces, you can start here: http://php.net/manual/en/language.namespaces.php ). In this case, the namespace would be AwesomePlugin\Inc.
Example:
File inc/helper.php would contain:
<?php namespace AwesomePlugin\Inc; class Helper { //....code here }
This way all the files in the inc folder will be compartmentalized, which will make them easier to use throughout your project. Especially if you are using a good autoloader! ( https://www.ndevr.io/blog/autoloader-and-wordpress/ ).
Note: You can easily create a default structure by using the wp-cli scaffold, see references for more info.
Conclusion
What was discussed in this article is, of course, not the only way of developing a plugin for a WordPress site, however, it gives the basic steps on how to properly structure one using the object-oriented programming approach. This, combined with other techniques, is a powerful and time-saver way to build performant software that will adapt to many situations.